해당 시리즈는 총 3부작으로 나누어져 있습니다.
- 실전에서 withRouter를 사용하기(1) :: Router 구성하기
- 실전에서 withRouter를 사용하기(2) :: styled-components를 활용하여 스타일링 적용하기
- 실전에서 withRouter를 사용하기(3) :: withRouter 사용하기
해당 시리즈에는 react의 styled-components에 대한 코드가 나올 것이다.
지금 우리는 withRouter
에 대해서 알아보는 과정이므로 styled-components에 대해서 잘 모르는 사람은 그냥 css 적용을 js 안에서 수행하구나~ 정도만 이해하고 넘어가도록 하자.
하지만 개인적인 추천으로 styled-components에 대해서 모르는 사람은 정말 멋지고 쿨한 기능이므로 해당 블로그의
styled-components 카테고리에서 사용 방법 및 Styled-Components의 철학에 대해서 조금 봐보는 것을 매우 강력히 추천한다.
결과적으로 우리는
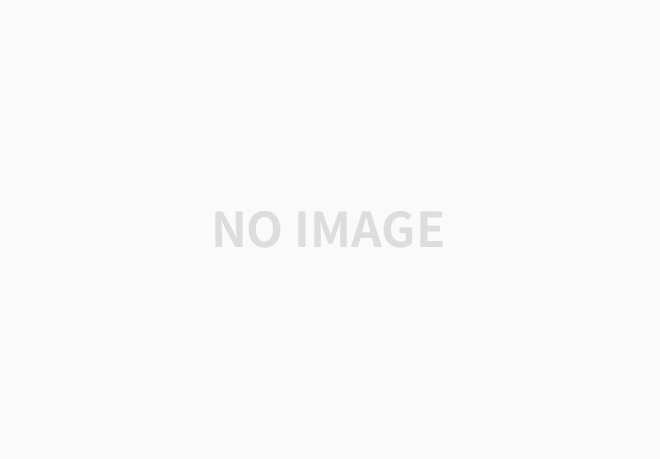
이런 웹 사이트의 상단 header를 구현할 것이고, 클릭하는 위치마다 border-bottom의 색이 변경될 것이다.
오늘은 style-components를 이용해 스타일링을 적용할 것이다.
지난 시간에 우리는 react-router-dom
을 이용해서 라우팅을 적용하였다.
그리고 다음과 같은 결과물이 탄생하였다.
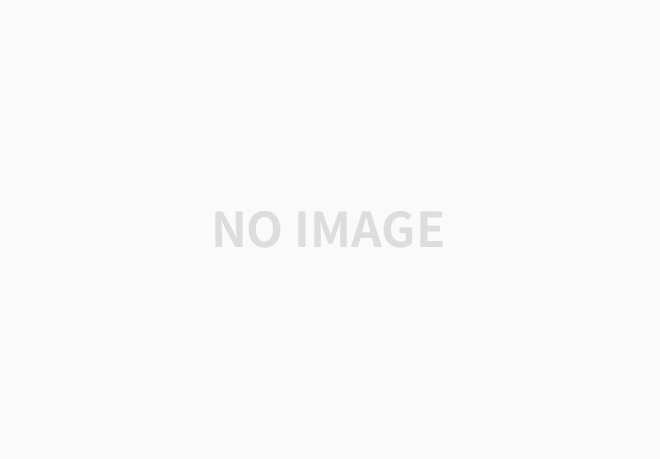
하지만 우리의 목표는 designbase.co.kr처럼 Navigation bar의 a태그를 클릭하면 해당 메뉴 아래에 파란 밑 줄이 생기는 것을 원하므로 스타일링을 간단하게 입혀보자.
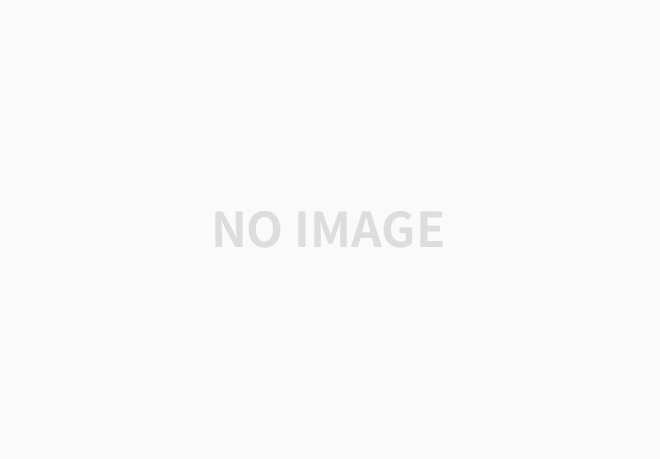
스타일링
일단 위에서 보다싶이 a태그이지만 a태그같이 보이지 않는다.
이는 styled-reset을 적용하여 GlobalStyle을 생성했기 때문이다.
다음과 같이 GlobalStyles.js를 생성해준다.
GlobalStyle.js 생성
import { createGlobalStyle } from "styled-components";
import reset from "styled-reset";
const GlobalStyles = createGlobalStyle`
${reset}
* {
box-sizing: border-box;
}
body {
font-size: 14px;
background-color: white;
font-family: -apple-system,system-ui,BlinkMacSystemFont,"Segoe UI",Roboto,"Helvetica Neue",Arial,sans-serif;
}
a {
text-decoration: none;
color: inherit;
cursor: pointer;
}
input, button {
background-color: transparent;
}
h1, h2, h3, h4, h5, h6 {
font-family:'Maven Pro', sans-serif;
}
`
export default GlobalStyles;
GlobalStyles.js 포함시키기
우리가 생성한 GlobalStyles.js를 App.js로 포함시켜주자.
import React from 'react';
import { BrowserRouter as Router } from "react-router-dom";
import { Header } from './components/Header';
import { Routes } from './components/Routes';
import GlobalStyles from "./components/GlobalStyles";
export const App = () => {
return (
<>
<GlobalStyles />
<Router>
<Header />
<Routes />
</Router>
</>
);
}
export default App;
그럼 css-reset을 React의 styled-components에서 할 수 있게 되었다.
그리고 해당 링크를 클릭했을 시에 효과를 주기 위해 상단 바 위치를 꾸며보자.
Header.js 스타일링
import React from 'react';
import { Link } from "react-router-dom";
import styled from "styled-components";
const SLink = styled(Link)`
padding: 15px 20px;
color: gray;
border-bottom: 4px solid #0050FF;
`;
const SItem = styled.li`
text-align: center;
`;
const SList = styled.ul`
display: flex;
`;
const SHeader = styled.header`
display: flex;
padding: 0px 30px;
width: 100%;
height: 55px;
align-items: center;
background-color: rgba(245, 245, 245, 0.8);
z-index: 10;
box-shadow: 0px 1px 3px 1px rgba(0, 0, 0, 0.2);
margin-bottom: 20px;
${SList}:first-child {
margin-right: auto;
}
`;
export const Header = () => {
return (
<SHeader>
<SList>
<SItem>
<SLink to="/">DESIGN BASE</SLink>
</SItem>
</SList>
<SList>
<SItem>
<SLink to="/about">소개</SLink>
</SItem>
</SList>
<SList>
<SItem>
<SLink to="/course-design">디자인 강좌</SLink>
</SItem>
</SList>
<SList>
<SItem>
<SLink to="/course-coding">웹코딩 강좌</SLink>
</SItem>
</SList>
<SList>
<SItem>
<SLink to="/bookmark">북마크</SLink>
</SItem>
</SList>
<SList>
<SItem>
<SLink to="/meetup">밋업</SLink>
</SItem>
</SList>
<SList>
<SItem>
<SLink to="/contact">문의하기</SLink>
</SItem>
</SList>
</SHeader>
);
}
그럼 다음과 같은 화면이 나오게 될 것이다.
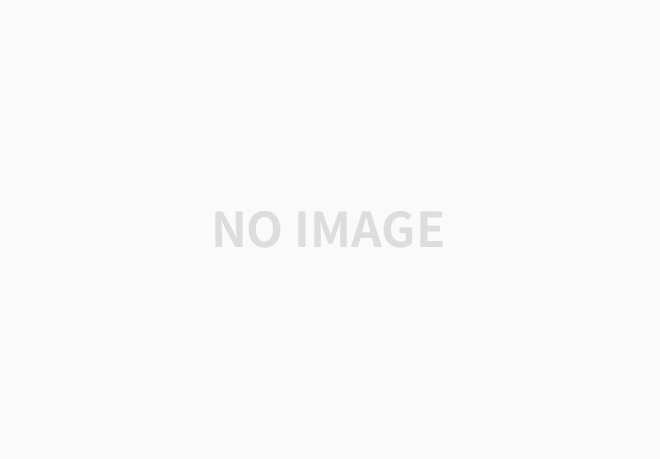
이제 클릭 했을 때 위치를 주기 위해 styled-components의 props를 이용해보자.
Props 이용하기
Header.js에 있는 SLink
컴포넌트에 props로 current
를 만들 것이다.
그리고 current props의 값이 false이면 border-bottom을 주지 않고 true면 border-bottom을 줘보자.
import React from 'react';
import { Link } from "react-router-dom";
import styled from "styled-components";
const SLink = styled(Link)`
padding: 15px 20px;
color: ${props => props.current ? "#0050FF" : "gray"};
border-bottom: 4px solid
${props => props.current ? "#0050FF" : "transparent"};
`;
... 생략
export const Header = () => {
return (
<SHeader>
<SList>
<SItem>
<SLink current={false} to="/">DESIGN BASE</SLink>
</SItem>
</SList>
<SList>
<SItem>
<SLink current={false} to="/about">소개</SLink>
</SItem>
</SList>
<SList>
<SItem>
<SLink current={false} to="/course-design">디자인 강좌</SLink>
</SItem>
</SList>
<SList>
<SItem>
<SLink current={false} to="/course-coding">웹코딩 강좌</SLink>
</SItem>
</SList>
<SList>
<SItem>
<SLink current={false} to="/bookmark">북마크</SLink>
</SItem>
</SList>
<SList>
<SItem>
<SLink current={false} to="/meetup">밋업</SLink>
</SItem>
</SList>
<SList>
<SItem>
<SLink current={false} to="/contact">문의하기</SLink>
</SItem>
</SList>
</SHeader>
);
}
그럼 아래와 같은 형태가 된다.
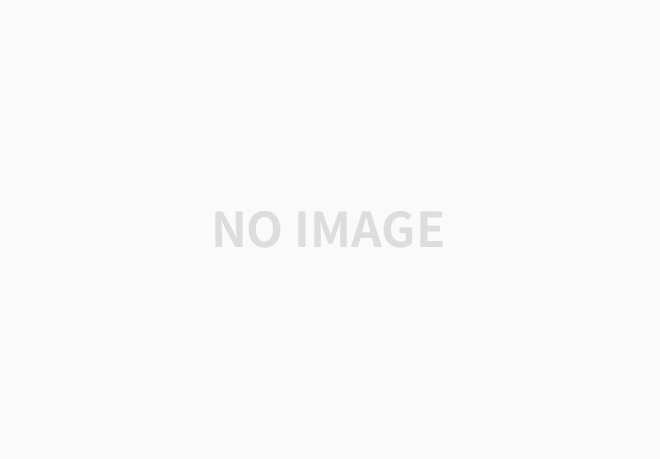
그리고 여기서 테스트를 위해 about 의 SLink
에 current
props를 true로 바꿔주면 우리가 예상한 대로 동작하게 된다.
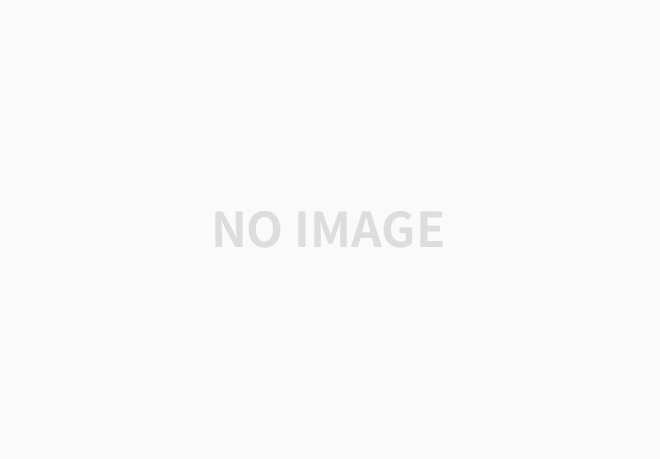
이제 다음 시간에는 이번 수업의 목적인 withRouter를 사용해 조건부 스타일링을 주고 마무리를 해보자.
댓글